In this short article let’s discuss how to create a rounded tab bar in Flutter instead default tab bar.
here is the default tab bar example code in Flutter.
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const RoundedTabBar(),
);
}
}
Here is the default tab bar widget code.
import 'package:flutter/material.dart';
class RoundedTabBar extends StatelessWidget {
const RoundedTabBar({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return DefaultTabController(
length: 4,
child: Scaffold(
appBar: AppBar(
title: const Text("Rounded AppBar Example"),
centerTitle: true,
),
body: Column(
children: const [
TabBar(
labelColor: Colors.black,
tabs: [
Tab(
text: "Tab 1",
),
Tab(
text: "Tab 2",
),
Tab(
text: "Tab 3",
),
Tab(
text: "Tab 4",
),
],
),
Expanded(
child: TabBarView(
children: [
Center(child: Text("View 1")),
Center(child: Text("View 2")),
Center(child: Text("View 3")),
Center(child: Text("View 4")),
],
),
)
],
),
),
);
}
}
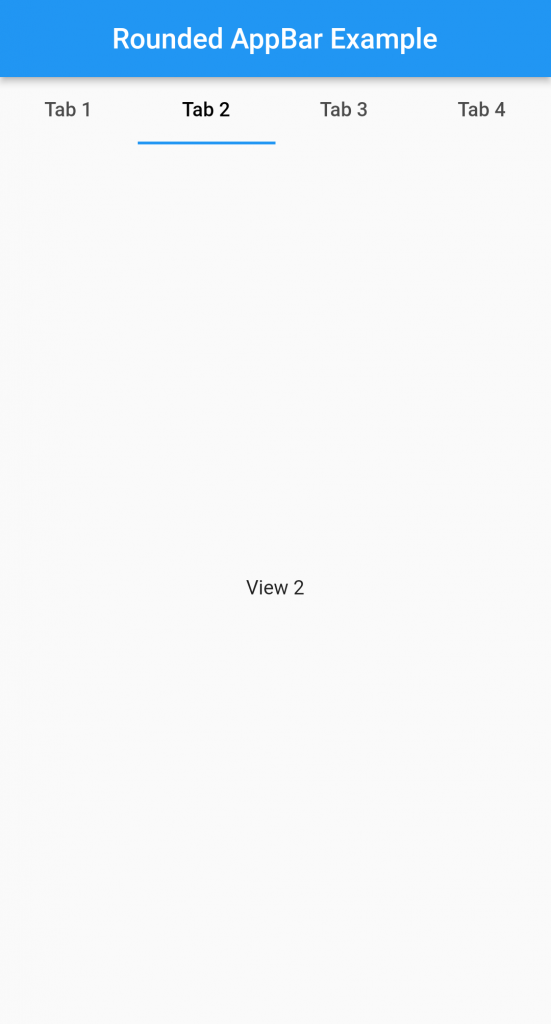
Now let’s discuss how to create a rounded tab bar in Flutter
Here is an example code for a rounded tab bar in Flutter.
import 'package:flutter/material.dart';
class RoundedTabBar extends StatelessWidget {
const RoundedTabBar({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return DefaultTabController(
length: 4,
child: Scaffold(
appBar: AppBar(
title: const Text("Rounded AppBar Example"),
centerTitle: true,
),
body: Column(
children: [
Container(
margin: const EdgeInsets.only(
top: 10.0,
),
height: 35.0,
child: TabBar(
labelColor: Colors.white,
unselectedLabelColor: Colors.black,
isScrollable: true,
indicator: BoxDecoration(
color: Colors.blue,
borderRadius: BorderRadius.circular(32),
),
tabs: const [
Tab(
text: "Tab 1",
),
Tab(
text: "Tab 2",
),
Tab(
text: "Tab 3",
),
Tab(
text: "Tab 4",
),
],
),
),
const Expanded(
child: TabBarView(
children: [
Center(child: Text("View 1")),
Center(child: Text("View 2")),
Center(child: Text("View 3")),
Center(child: Text("View 4")),
],
),
)
],
),
),
);
}
}
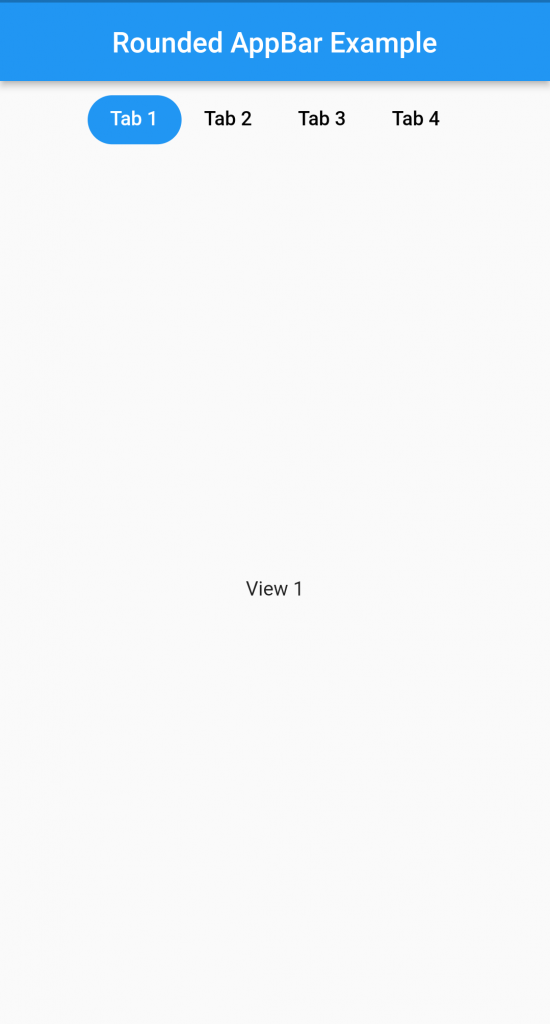
Read official Tab Bar Documentation
Learn more: