Table of Contents
Hi guys, in this article, we will discuss 40 Useful Flutter extension methods that you never knew.
What are extension methods in Flutter?
An extension method in Flutter is a way to add new functionality to an existing class without modifying its original source code. This is achieved by creating a new class (also known as an “extension”) that “extends” the functionality of the original class.
The syntax for an extension method is to use the extension
keyword, followed by the name of the extension class and the on
keyword, followed by the class that you want to extend. Inside the extension class, you can define new methods, properties, and operators that can be used on instances of the original class.
1. Randomly shuffles the elements in a list.
extension ListShuffle on List {
void shuffle() {
var random = new Random();
for (var i = this.length - 1; i > 0; i--) {
var n = random.nextInt(i + 1);
var temp = this[i];
this[i] = this[n];
this[n] = temp;
}
}
}
2. Swaps the elements at indices i and j in a list.
extension ListSwap on List {
void swap(int i, int j) {
var temp = this[i];
this[i] = this[j];
this[j] = temp;
}
}
3. Reverses the order of the characters in a string.
extension StringReverse on String {
String reverse() {
return this.split('').reversed.join();
}
}
4. Determines whether a string is a palindrome (a word or phrase that reads the same backwards as forwards)
extension StringPalindrome on String {
bool isPalindrome() {
return this == this.split('').reversed.join();
}
}
5. Counts the number of vowels in a string.
extension StringVowels on String {
int countVowels() {
var vowels = 'aeiouAEIOU';
return this.split('').where((c) => vowels.contains(c)).length;
}
}
6. Counts the number of words in a string.
extension StringWords on String {
int countWords() {
var words = this.split(RegExp(r'\s+'));
return words.where((w) => w.isNotEmpty).length;
}
}
7. Determines whether a string is a valid email address.
extension StringEmail on String {
bool isEmail() {
return RegExp(r"^[a-zA-Z0-9.a-zA-Z0-9.!#$%&'*+-/=?^_`{|}~]+@[a-zA-Z0-9]+\.[a-zA-Z]+").hasMatch(this);
}
}
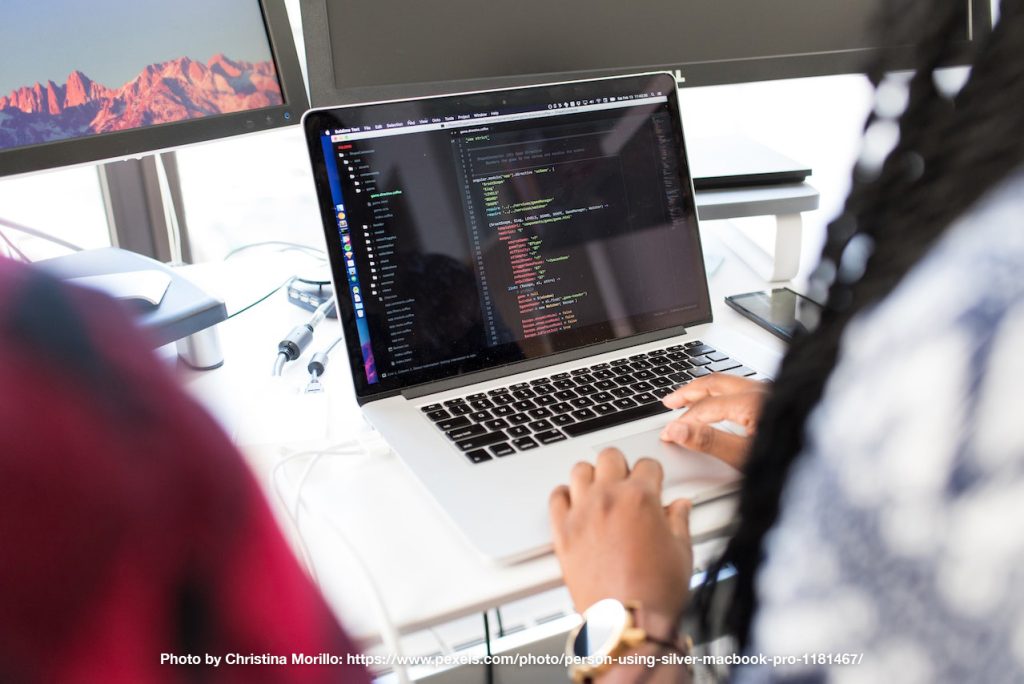
8. Determines whether a string is a valid phone number. (US phone numbers)
extension StringPhoneNumber on String {
bool isPhoneNumber() {
// regular expression to match phone number
final phoneNumberRegex = RegExp(r'^(?:(?:\+?1\s*(?:[.-]\s*)?)?(?:\(\s*([2-9]1[02-9]|[2-9][02-8]1|[2-9][02-8][02-9])\s*\)|([2-9]1[02-9]|[2-9][02-8]1|[2-9][02-8][02-9]))\s*(?:[.-]\s*)?)?([2-9]1[02-9]|[2-9][02-9]1|[2-9][02-9]{2})\s*(?:[.-]\s*)?([0-9]{4})(?:\s*(?:#|x\.?|ext\.?|extension)\s*(\d+))?$');
return phoneNumberRegex.hasMatch(this);
}
}
9. Determines whether a string contains only numeric characters.
extension StringNumeric on String {
bool isNumeric() {
return RegExp(r'^[0-9]+$').hasMatch(this);
}
}
10. Determines whether a string contains only alphabetic characters.
extension StringAlpha on String {
bool isAlpha() {
return RegExp(r'^[a-zA-Z]+$').hasMatch(this);
}
}
11. Determines whether a string contains only alphanumeric characters.
extension StringAlphanumeric on String {
bool isAlphanumeric() {
return RegExp(r'^[a-zA-Z0-9]+$').hasMatch(this);
}
}
Do you know What is the Flutter?
12. Returns the age of a person based on their birthdate.
extension DateTimeAge on DateTime {
int age() {
DateTime current = DateTime.now();
int age = current.year - this.year;
if (current.month < this.month || (current.month == this.month && current.day < this.day)) {
age--;
}
return age;
}
}
13. Determines whether a DateTime object represents today’s date.
extension DateTimeToday on DateTime {
bool isToday() {
DateTime now = DateTime.now();
return this.year == now.year && this.month == now.month && this.day == now.day;
}
}
14. Determines whether a DateTime object represents yesterday’s date.
extension DateTimeYesterday on DateTime {
bool isYesterday() {
DateTime yesterday = DateTime.now().subtract(Duration(days: 1));
return this.year == yesterday.year && this.month == yesterday.month && this.day == yesterday.day;
}
}
15. Determines whether a DateTime object represents a date in the future.
extension DateTimeFuture on DateTime {
bool isFuture() {
return this.isAfter(DateTime.now());
}
}
16. Determines whether a DateTime object represents a date in the past.
extension DateTimePast on DateTime {
bool isPast() {
return this.isBefore(DateTime.now());
}
}
17. Determines whether the year of a DateTime object is a leap year.
extension DateTimeLeapYear on DateTime {
bool isLeapYear() {
int year = this.year;
if (year % 4 != 0) {
return false;
} else if (year % 100 != 0) {
return true;
} else if (year % 400 != 0) {
return false;
} else {
return true;
}
}
}
18. Returns the number of days in the month of a DateTime object.
extension DateTimeDaysInMonth on DateTime {
int daysInMonth() {
int year = this.year;
int month = this.month;
if (month == 2) {
if (this.isLeapYear()) {
return 29;
} else {
return 28;
}
} else if (month == 4 || month == 6 || month == 9 || month == 11) {
return 30;
} else {
return 31;
}
}
}
19. Returns the day of the week of a DateTime object as a string (e.g. “Monday”).
extension DateTimeWeekday on DateTime {
String weekday() {
int day = this.weekday;
switch (day) {
case 1:
return 'Monday';
case 2:
return 'Tuesday';
case 3:
return 'Wednesday';
case 4:
return 'Thursday';
case 5:
return 'Friday';
case 6:
return 'Saturday';
case 7:
return 'Sunday';
default:
return '';
}
}
}
Here are the other useful flutter extension methods that can use for your mobile app development process.
20. Returns a string describing the time elapsed since a DateTime object (e.g. “3 minutes ago”).
extension DateTimeTimeAgo on DateTime {
String timeAgo() {
Duration duration = DateTime.now().difference(this);
if (duration.inDays >= 365) {
int years = (duration.inDays / 365).floor();
return '$years year${years > 1 ? 's' : ''} ago';
}
if (duration.inDays >= 30) {
int months = (duration.inDays / 30).floor();
return '$months month${months > 1 ? 's' : ''} ago';
}
if (duration.inDays > 0) {
return '${duration.inDays} day${duration.inDays > 1 ? 's' : ''} ago';
}
if (duration.inHours > 0) {
return '${duration.inHours} hour${duration.inHours > 1 ? 's' : ''} ago';
}
if (duration.inMinutes > 0) {
return '${duration.inMinutes} minute${duration.inMinutes > 1 ? 's' : ''} ago';
}
if (duration.inSeconds > 0) {
return '${duration.inSeconds} second${duration.inSeconds > 1 ? 's' : ''} ago';
}
return 'Just now';
}
}
21. Check whether the list is sorted or not.
extension ListSorted on List {
bool isSorted() {
for (int i = 0; i < this.length - 1; i++) {
if (this[i] > this[i + 1]) {
return false;
}
}
return true;
}
}
22. Returns the maximum element in the list.
extension ListMax on List {
dynamic max() {
return this.reduce(max);
}
}
23. Returns the minimum element in the list.
extension ListMin on List {
dynamic min() {
return this.reduce(min);
}
}
24. Returns the sum of all the elements in the list.
extension ListSum on List<num> {
num sum() {
return this.reduce((value, element) => value + element);
}
}
25. Returns the average of all the elements in the list.
extension ListAverage on List<num> {
double average() {
return this.isNotEmpty ? this.sum() / this.length : 0;
}
}
26. Returns a new list with unique elements.
extension ListUnique on List {
List unique() {
return this.toSet().toList();
}
}
27. Returns a new list with the common elements between the current and other list.
extension ListIntersection on List {
List intersection(List otherList) {
return this.where((element) => otherList.contains(element)).toList();
}
}
28. Returns a new list with the elements from the current list that are not in the other list.
extension ListDifference on List {
List difference(List otherList) {
return this.where((element) => !otherList.contains(element)).toList();
}
}
29. Returns a random element from the list.
extension ListRandom on List {
dynamic random({int seed}) {
var random = new Random(seed);
return this[random.nextInt(this.length)];
}
}
30. Returns the count of a specific element in the list.
extension ListCount on List {
int count(var element) {
int count = 0;
for (var item in this) {
if (item == element) {
count++;
}
}
return count;
}
}
31. Check whether the list contains a specific element or not.
extension ListContains on List {
bool contains(var element) {
return this.any((e) => e == element);
}
}
32. Check whether the string is in upper case or not.
extension StringUpper on String {
bool isUpper() {
return this == this.toUpperCase();
}
}
33. Check whether the string is in lowercase or not.
extension StringLower on String {
bool isLower() {
return this == this.toLowerCase();
}
}
34. Returns a new string with the first letter capitalized.
extension StringCapitalize on String {
String capitalize() {
return "${this[0].toUpperCase()}${this.substring(1)}";
}
}
35. Returns a list of words from a string.
extension StringWords on String {
List<String> words() {
return this.split(" ");
}
}
Is that easy to understand Flutter extension methods?
36. returns a list of lines from a string.
extension StringLines on String {
List<String> lines() {
return this.split("\n");
}
}
37. Check whether the string contains only ASCII characters or not.
extension StringAscii on String {
bool isAscii() {
return RegExp(r'^[\x00-\x7F]+$').hasMatch(this);
}
}
38. Truncates a string to a specified maximum length.
extension StringTruncate on String {
String truncate(int maxLength) {
return this.length > maxLength ? "${this.substring(0, maxLength)}..." : this;
}
}
39. Replaces all occurrences of a set of characters with their corresponding replacements.
extension StringReplaceAll on String {
String replaceAll(Map<String, String> replacements) {
String newString = this;
replacements.forEach((key, value) {
newString = newString.replaceAll(key, value);
});
return newString;
}
}
40. Check whether a DateTime object represents a weekend day or not.
extension DateTimeWeekend on DateTime {
bool isWeekend() {
return this.weekday == DateTime.saturday || this.weekday == DateTime.sunday;
}
}
Conclusion
In this article, we discussed 40 useful flutter extension methods that can use in your mobile app journey. See you in the next article. Have a nice day.
Learn more:
How to use Quick alert package